How to create a secure login and registration system in PHP and MYSQL database
Complete secure login and registration system with source code. This code also Prevents MySQL injection. Learn how to create this page with the steps we have listed. A video is also provided for easy learning. First, Go to your PHPMyAdmin and create a new database.
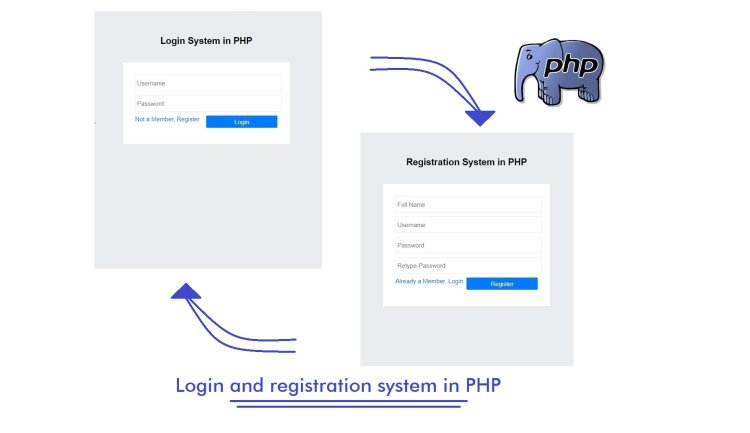
Table of content
- About the secure login and registration system in PHP
- Features of the secure login and registration system in PHP
- Script folder includes
- how to create a secure login and registration system in PHP
- Video Demo
- How to downloaded and run the source code
- The screenshots
About the secure login and registration system in PHP
The project called the secure login and registration system in PHP is a secure Login system and registration system, which can be used to create a login and registration page for your website.
Any website that wants people to register or be part of their community needs a login and registration page, not just a registration or login page but a secure one that will help in preventing malicious attacks to your website like MySQL injection. This login and registration system in written in PHP and mysql.
Features of the secure login and registration system in PHP
- Login system
- Registration system
- Logout system
- Prevents MYSQL injection
- Good interface
- Responsive design
- Uses PDO
- Displays all error messages
Script folder Includes
- PHP
- MYSQL Database
- CSS
- HTML
How to create the secure login and registration system in PHP
Create a database
In other to store and retrieve our registered users, we need to create a database (MYSQL Database). A hosting server is also required to do this. We will be using XAMPP in this post. A video demonstration is provided in this page for easy learning.
- Open XAMPP and start Apache and MYSQL.
- Open your Browser and Go to localhost/phpmyadmin/
- Click on New.
- Input the database name called login_registration_system and click on create.
Creating the database tables
- After the database has been created, input Users and select number of columns to be 4 then click on Go.
- Input and select as seen in the image below and click Save.
Connecting to the database
You need to connect to the database for you to be able to store and get information about the registered user.
- Go to [Your local disk]/xampp/htdocs directory and create a folder called login-registration-system.
- Go to [Your local disk]/xampp/htdocs/login-registration-system
- Create a
config.php
file inside the folder "login-registration-system" - Copy the code below and paste into it.
CONFIG.PHP
<?php
define( 'DOMAIN', 'http://localhost/login-registration-system/');
$host_name = "localhost";
$username = "root";
$password = "";
$db_name = "login_registration_system";
try {
$conn = new PDO("mysql:host=$host_name; dbname=$db_name", $username, $password);
$conn->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
} catch (PDOException $e) {
echo "connection failed : ". $e->getMessage();
}
Creating the registration Form
Then the next thing is to create the registration page.
- Create a file called
register.php
and save inside the same folder with the config.php. - Copy the code below and paste inside the
register.php
file.
REGISTER.PHP
<?php
session_start();
require 'config.php';
// this returns back the form details if there was an error
$fullname = $_SESSION['registration-data']['fullname'] ?? null;
$username = $_SESSION['registration-data']['username'] ?? null;
$password = $_SESSION['registration-data']['password'] ?? null;
$rpassword = $_SESSION['registration-data']['rpassword'] ?? null;
// delete the registration session
unset($_SESSION['registration-data']);
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="style.css">
<title>Login and Registration system in PHP</title>
</head>
<body>
<h2>Registration System in PHP</h2>
<form action="register_process.php" method="post">
<!-- error message -->
<?php
if (isset($_SESSION['register_error'])) : ?>
<p style="color: red; text-align: center;"><?= $_SESSION['register_error']; unset($_SESSION['register_error']);?></p><br>
<?php endif ?>
<!-- /error message -->
<input type="text" value="<?= $fullname ?>" name="fullname" placeholder="Full Name"><br>
<input type="text" name="username" value="<?= $username ?>" placeholder="Username"><br>
<input type="password" name="password" value="<?= $password ?>" placeholder="Password"><br>
<input type="password" name="rpassword" value="<?= $rpassword ?>" placeholder="Retype-Password"><br>
<input type="submit" name="submit" value="Register">
<a href="login.php">Already a Member, Login</a>
</form>
</body>
</html>
Creating the CSS file
Lets design it by creating the CSS file.
- Create a file called
style.
css
and save inside the folder with the config and register files. - Copy the CSS codes below and paste into it
STYLE.CSS
*{
margin: 0;
}
body{
background-color: #e9ecef;
font-family: Arial, Helvetica, sans-serif;
display: flex;
justify-content: center;
align-items: center;
flex-direction: column;
font-size: 15px;
}
h2{
margin-top: 60px ;
margin-bottom: 40px ;
}
form{
background-color: white;
padding: 30px;
width: 350px;
}
input{
height: 30px;
width:100%;
margin-bottom: 10px;
border-radius: 2px;
border:1px solid #e9eced;
font-size: 15px;
padding: 4px;
}
input:focus {
border:1px solid #e9eced;
}
a{
text-decoration: none;
color: #007bff;
}
[type="submit"]{
background-color: #007bff;
color: white;
width:50%;
border:none;
cursor: pointer;
float: right;
}
Creating the login Form
Then create the login login page.
- Create a file called
login.php
and save inside the folder with the others - Copy the code below and paste in it.
LOGIN.PHP
<?php
session_start();
require 'config.php';
// this returns back the form details if there was an error
$username = $_SESSION['login-data']['username'] ?? null;
$password = $_SESSION['login-data']['password'] ?? null;
// delete the registration session
unset($_SESSION['login-data']);
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="style.css">
<title>Login and Registration system in PHP</title>
</head>
<body>
<h2>Login System in PHP</h2>
<form action="login_process.php" method="post">
<!-- error message -->
<?php
if (isset($_SESSION['register_success'])) : ?>
<p style="color: green; text-align: center"><?= $_SESSION['register_success']; unset($_SESSION['register_success']);?></p><br>
<?php elseif (isset($_SESSION['login_error'])) : ?>
<p style="color: red; text-align: center;"><?= $_SESSION['login_error']; unset($_SESSION['login_error']);?></p><br>
<?php endif ?>
<!--/ error message -->
<input type="text" value="<?= $username ?>" name="username" placeholder="Username"><br>
<input type="password" value="<?= $password ?>" name="password" placeholder="Password"><br>
<input type="submit" name="submit" value="Login">
<a href="register.php">Not a Member, Register</a>
</form>
</body>
</html>
Creating the registration process
We need to create a page that will process the registration form and make sure the person registering is not injecting any MYSQL codes. This is where we validate the form before inserting into the database.
- Create a file called
register_process.php
and save. - Copy the code below and paste in it.
REGISTER_PROCESS.PHP
<?php
session_start();
require 'config.php';
if(isset($_POST['submit'])){
$fullname = filter_var($_POST['fullname'], FILTER_SANITIZE_FULL_SPECIAL_CHARS);
$username = filter_var($_POST['username'], FILTER_SANITIZE_FULL_SPECIAL_CHARS);
$password = filter_var($_POST['password'], FILTER_SANITIZE_FULL_SPECIAL_CHARS);
$rpassword = filter_var($_POST['rpassword'], FILTER_SANITIZE_FULL_SPECIAL_CHARS);
if(!$fullname){
$_SESSION['register_error'] = "Full Name is required" ;
}elseif (!$username) {
$_SESSION['register_error'] = "Username is required";
}elseif(!$password){
$_SESSION['register_error'] = "password is required";
}elseif(!$rpassword){
$_SESSION['register_error'] = "Confirm Password is required";
}elseif(strlen($password) < 8 || strlen($rpassword) < 8){
$_SESSION['register_error'] = "Password or Confirm password must be 8+ characters";
}elseif($password !== $rpassword){
$_SESSION['register_error'] = "Passwords do not match";
}else{
//hash password
$hash_password = password_hash($password, PASSWORD_DEFAULT);
// check if username or email already exists
$statement = $conn->prepare("SELECT * FROM users WHERE username= '$username' ");
$statement->execute();
$statement->fetchAll(PDO::FETCH_ASSOC);
$get_username = $statement->rowcount();
if ($get_username > 0) {
$_SESSION['register_error'] = "Username already exist";
}
}
// redirect to register page if error
if (isset($_SESSION['register_error'])) {
//pass form back to register page
$_SESSION['registration-data'] = $_POST;
header('location: ' . DOMAIN . 'register.php');
die();
}else{
try{
//insert into users table
$insert_user_query = $conn->prepare("INSERT INTO users (fullname, username, password)
VALUES(:fullname, :username, :hash_password)");
$insert_user_query->bindValue(':fullname', $fullname);
$insert_user_query->bindValue(':username', $username);
$insert_user_query->bindValue(':hash_password', $hash_password);
$insert_user_query->execute();
// redirect to login page with sucess message
$_SESSION['register_success'] = "Successfully Registered. please login";
header('location: ' . DOMAIN . 'login.php');
die();
} catch (PDOException $err) {
$_SESSION['register_error'] = "Error: " . $err->getMessage();
header('location: ' . DOMAIN . 'register.php');
die();
}
}
}else{
//if button was not clicked
header('location: ' . DOMAIN . 'register.php');
exit();
}
Creating the login process
We also need to create a page that will process the login form and make sure the person trying to login is registered or is in the database.
- Create a file called
login_process.php
and save. - Copy the code below and paste into it.
LOGIN_PROCESS.PHP
<?php
session_start();
require 'config.php';
if(isset($_POST['submit'])){
$username = filter_var($_POST['username'], FILTER_SANITIZE_FULL_SPECIAL_CHARS);
$password = filter_var($_POST['password'], FILTER_SANITIZE_FULL_SPECIAL_CHARS);
if (!$username) {
$_SESSION['login_error'] = "Username is required";
}elseif(!$password){
$_SESSION['login_error'] = "password is required";
}else{
//get user information from database
$statement = $conn->prepare('SELECT * FROM users WHERE username = :username ');
$statement->bindValue(':username', $username);
$statement->execute();
$statement_result = $statement->rowcount();
if ($statement_result === 1) {
//convert record to an array
$get_user = $statement->fetch(PDO::FETCH_ASSOC);
$database_password = $get_user['password'];
//compare form password wth database password
if (password_verify($password, $database_password)) {
// set session for access control
$_SESSION['user-id-session'] = $get_user['id'];
//log the person in
header('location: ' . DOMAIN . 'index.php');
}else {
$_SESSION['login_error'] = "Incorrect Username or Password";
}
}else {
$_SESSION['login_error'] = "User not found";
}
}
// redirect to login page if error
if (isset($_SESSION['login_error'])) {
//pass form back to login page
$_SESSION['login-data'] = $_POST;
header('location: ' . DOMAIN . 'login.php');
exit();
}
}else{
//if button was not clicked
header('location: ' . DOMAIN . 'login.php');
exit();
}
Creating the index page
Let’s create a home page that the user is redirected to if login was successful.
- Create a file called
index.php
and save. - Copy the code below and paste in it.
INDEX.PHP
<?php
session_start();
require 'config.php';
// get current user from database
if (isset($_SESSION['user-id-session'])) {
$id = filter_var($_SESSION['user-id-session'], FILTER_SANITIZE_NUMBER_INT);
$statement = $conn->prepare('SELECT * FROM users WHERE id = :id ');
$statement->bindValue(':id', $id);
$statement->execute();
$statement_result = $statement->fetch(PDO::FETCH_ASSOC);
}else{
header('location: ' . DOMAIN . 'login.php');
exit();
}
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="style.css">
<title>Home</title>
</head>
<body>
<h2> Hello <?php echo $statement_result['fullname'] ?> </h2>
<a href="logout.php">logout</a>
</body>
</html>
Creating the logout system
Let create a logout button that allows the user to logout if they want to logout.
- Create a file called
logout.php
and save. - Copy the code below and paste in it.
LOGOUT.PHP
<?php
session_start();
require 'config.php';
//destroy all session and redirect user to home page
session_destroy();
header('location: ' . DOMAIN . 'login.php' );
die();
NOTE: ALL THE PAGES ARE REQUIRED, AS THEY ALL WORK TOGETHER. ANY MISSING PAGE MIGHT RESULT TO ERRORS.
Video Demo
Video demonstration is provided for easy learning. Watch video.
How to downloaded and run the source code
You can download the zipped source code if you like. The zipped source code is attached below.
- Go to the attached zipped file which is below.
- Click on the attached zipped file to start downloading.
- Go to downloads folder on your computer and unzip/ extract it.
- Copy the extracted folder and paste in [Your local disk]/xampp/htdocs directory.
- Go to your browser and open localhost/login-registration-system.
- You can open and edit on your code/ text editor.